Java Programming
Basic coding skills
Declare a main method
public static void main(String[] args) { statements }
- A method is a block of code that performs a task.
- Every Java application contains one main method. You can code the main method declaration as shown. The statements within the braces are run when the program is executed.
public class InvoiceApp
{
public static void main(String[] args)}
{ // begin main method
System.out.println("Welcome to the Invoice Total Calculator");} // end main method
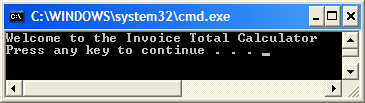
- The public keyword means that other classes can access the main method.
- The static keyword means that the method can be called directly from the other classes without first creating an object.
- The void keyword means that the method won't return any values.
- The main identifier is the name of the method. When you code a method, always include parentheses after the name of the method.
- The code in the parentheses lists the arguments that the method uses. Every main method receives an argument named args.